reduceRight
reduceRightメソッドは、
Arrayオブジェクト内の隣同士の要素を右から左へ処理して単一の要素にして順番の処理をしていく。
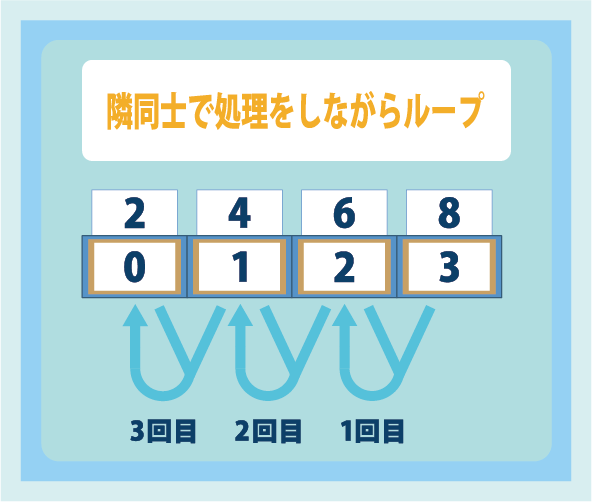
最後のループの戻り値 = 配列.reduceRight ( function (totalValue, currentValue, index, array) {
return 処理の結果(totalValueに格納);
}, initValue);
[引数の意味] ========================
totalValue : 前のループの戻り値(initValueがない場合は配列の最初の値)
currentValue : 配列の値が1つずつ渡される
index : 配列のキーが1つずつ渡される
array : 配列が渡される
initValue : 配列の最初の値(初回のtotalValue値になる)
initValueなし
let ary = [1, 2, 3, 4, 5];
let result = ary.reduceRight(function(totalValue, currentValue, index, array) {
console.log("totalValue : " + totalValue);
console.log("currentValue : " + currentValue);
console.log("index : " + index);
console.log("array : " + array);
console.log("============================= ");
return totalValue + currentValue;
});
console.log("result : " + result);
totalValue : 5
currentValue : 4
index : 3
array : 1,2,3,4,5
=============================
totalValue : 9
currentValue : 3
index : 2
array : 1,2,3,4,5
=============================
totalValue : 12
currentValue : 2
index : 1
array : 1,2,3,4,5
=============================
totalValue : 14
currentValue : 1
index : 0
array : 1,2,3,4,5
=============================
result : 15
initValueあり
let ary = [1, 2, 3, 4, 5];
let result = ary.reduceRight(function(totalValue, currentValue, index, array) {
console.log("totalValue : " + totalValue);
console.log("currentValue : " + currentValue);
console.log("index : " + index);
console.log("array : " + array);
console.log("============================= ");
return totalValue + currentValue;
}, 10);
console.log("result : " + result);
totalValue : 10
currentValue : 5
index : 4
array : 1,2,3,4,5
=============================
totalValue : 15
currentValue : 4
index : 3
array : 1,2,3,4,5
=============================
totalValue : 19
currentValue : 3
index : 2
array : 1,2,3,4,5
=============================
totalValue : 22
currentValue : 2
index : 1
array : 1,2,3,4,5
=============================
totalValue : 24
currentValue : 1
index : 0
array : 1,2,3,4,5
=============================
result : 25
アロー関数
最後のループの戻り値 = 配列.reduceRight ( ( totalValue, currentValue, index, array ) => 処理 )
let ary = [1, 2, 3, 4, 5];
const result = ary.reduceRight((totalValue, currentValue) => totalValue + currentValue);
console.log("result : " + result);
result : 15
コールバック関数
コールバック関数
最後のループの戻り値 = 配列.reduceRight ( reducer )
let ary = [1, 2, 3, 4, 5];
// コールバック関数
function reducer(totalValue, currentValue){
return totalValue + currentValue;
}
const result = ary.reduceRight(reducer);
console.log("result : " + result);
result : 15