v-bind:class
v-bind:classは、スタイルクラスを要素に割り当てるために利用します。
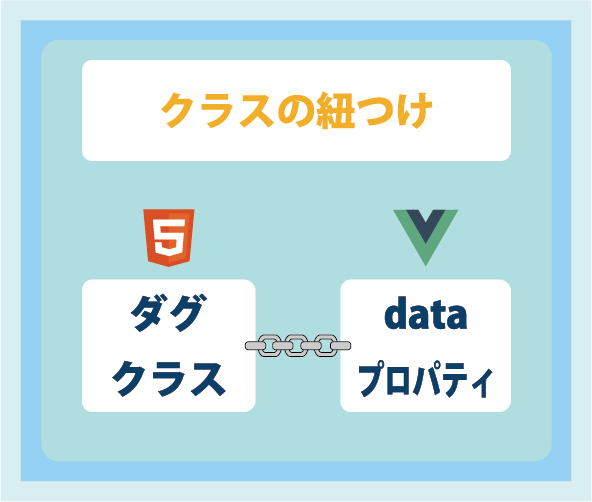
[ HTML ] =============================
<要素名 v-bind:class=”{ クラス名 }”>
<要素名 :class=”{ クラス名 }”>※省略時
[ Vue.js ] =============================
data() {
return {
データプロパティ名 : true;
};
}
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-bind:classについて</title>
</head>
<body>
<div id="app">
<p :class="'example'">こんにちは!</p>
</div>
<script src="./js/class.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
example: true,
};
},
}).mount("#app");
.example {
background-color: cornflowerblue;
color: white;
}
こんにちは!
文字列配列
バインドされたスタイルクラスは、スタイルクラス名を文字列の配列として受け渡す事もできます。
[ HTML ] =============================
<要素名 v-bind:class=”[ データプロパティ名 ]”>
<要素名 :class=”[ データプロパティ名 ]”>※省略時
[ Vue.js ] =============================
data() {
return {
データプロパティ名 : クラス名;
};
}
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-bind:class(文字列配列)について</title>
</head>
<body>
<div id="app">
<p :class="[ colorClass, frameClass ]">こんにちは!</p>
</div>
<script src="./js/class_str.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
colorClass: "color",
frameClass: "frame",
};
},
}).mount("#app");
.color {
background-color: cornflowerblue;
color: white;
}
.frame {
border: 2px solid black;
padding: 1rem;
}
こんにちは!
動的にクラスを変更1
バインドされたスタイルクラスは、Boolean型で動的に切り替える事ができます。
[ HTML ] =============================
<要素名 v-bind:class=”{ クラス名 : データプロパティ名 }”>
<要素名 :class=”{ クラス名 : データプロパティ名 }”>※省略時
[ Vue.js ] =============================
data() {
return {
データプロパティ名 : Boolean型;
};
}
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-bind:class(動的)について</title>
</head>
<body>
<div id="app">
<p :class="{ text : isActive }">こんにちは!</p>
<button @click="isActive = !isActive">ボタン</button>
</div>
<script src="./js/dynamic.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
isActive: true,
};
},
}).mount("#app");
.text{
background-color: cornflowerblue;
color: white;
}
こんにちは!
動的にクラスを変更2
スタイルクラスを動的に変更する方法は、computedを使用します。
[ HTML ] =============================
<要素名 v-bind:class=”{ computedのプロパティ名 }”>
<要素名 :class=”{ computedのプロパティ名 }”>※省略時
[ Vue.js ] =============================
data() {
return {
データプロパティ名 : Boolean型;
};
},
computed: {
textObject: function () {
return {
text: this.データプロパティ名,
frame: this.データプロパティ名
};
},
},
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-bind:class(動的2)について</title>
</head>
<body>
<div id="app">
<p :class="textObject">こんにちは!</p>
<button @click="isActive = !isActive">ボタン</button>
</div>
<script src="./js/dynamic.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
isActive: true,
};
},
computed: {
textObject: function () {
return {
text: this.isActive,
frame: this.isActive,
};
},
},
}).mount("#app");
.text {
background-color: cornflowerblue;
color: white;
}
.frame {
border: 2px solid black;
padding: 1rem;
margin-bottom: 0.5rem;
}
こんにちは!