from
fromは、配列風オブジェクトや反復可能オブジェクトから配列を作成します。
※配列風オブジェクトは、配列に似てますが配列用のメソッドは使用できません
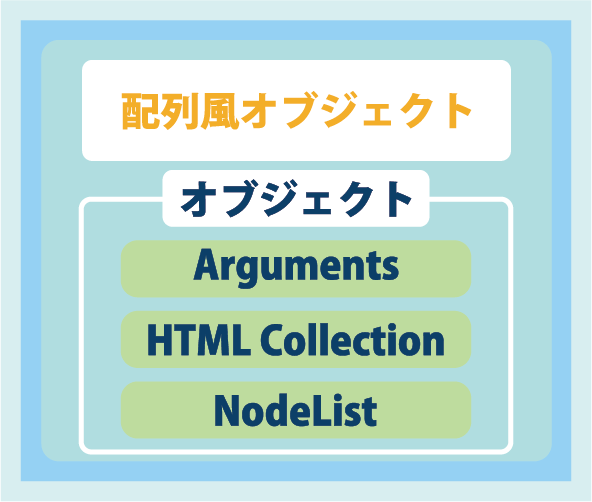
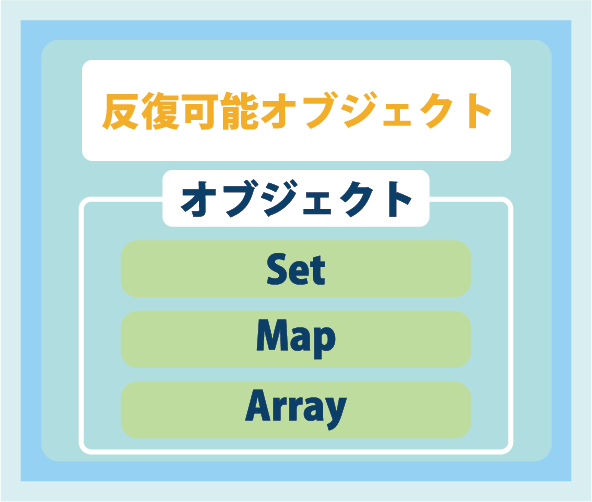
Argumentから配列生成
Argumentsは、関数内で使用可能な配列風オブジェクトになります。
function add(){
console.log("arguments[0] : " + arguments[0])
console.log("arguments[1] : " + arguments[1])
console.log("arguments[2] : " + arguments[2])
console.log("form : " + Array.from(arguments))
}
add(10,20,30)
arguments[0] : 10
arguments[1] : 20
arguments[2] : 30
form : 10,20,30
HTML Collectionから配列生成
HTML Collectionは、HTMLタグのみを取得したElementになります。
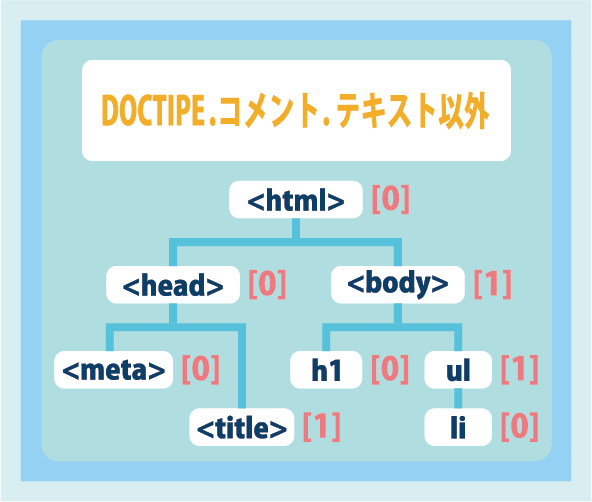
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- コメント123 -->
<h1>確認用</h1>
<ul class="ul-block">
<li class="list_item">menu1</li>
<li class="list_item">menu2</li>
<li class="list_item">menu3</li>
</ul>
<script src="./main.js"></script>
</body>
</html>
console.dir(document.children)
console.dir(document.children[0].children)
console.dir(document.children[0].children[0].children)
console.dir(document.children[0].children[1].children)
console.dir(document.children[0].children[1].children[1].children)
const elements = document.getElementsByClassName( "ul-block" )
const elementsArray = Array.from( elements )
// スプレッド構文(展開)
console.log(...elementsArray)
HTMLCollection(1)
0: html
length: 1
[[Prototype]]: HTMLCollection
HTMLCollection(2)
0: head
1: body
length: 2
[[Prototype]]: HTMLCollection
HTMLCollection(4)
0: meta
1: meta
2: meta
3: title
viewport: meta
length: 4
[[Prototype]]: HTMLCollection
HTMLCollection(3)
0: h1
1: ul.ul-block
2: script
length: 3
[[Prototype]]: HTMLCollection
HTMLCollection(3)
0: li.list_item
1: li.list_item
2: li.list_item
length: 3
[[Prototype]]: HTMLCollection
<ul class=”ul-block”>
<li class=”list_item”>
::marker
”menu1″
</li>
<li class=”list_item”>
::marker
”menu2″
</li>
<li class=”list_item”>
::marker
”menu3″
</li>
</ul>
NodeListから配列生成
NodeListは、テキストやコメント・HTMLタグなどが配列風オブジェクトに格納されています。
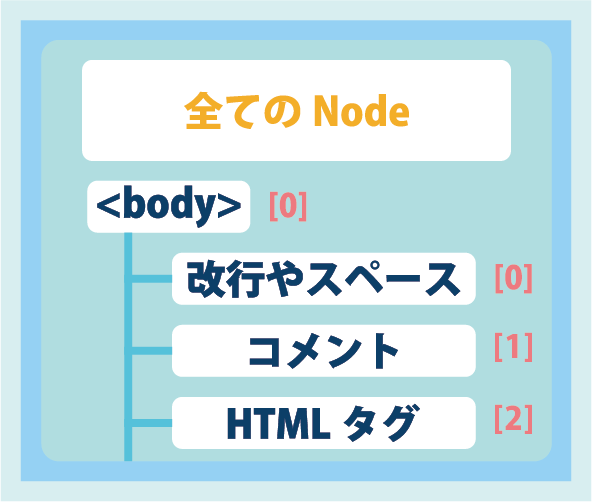
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- コメント123 -->
<h1>確認用</h1>
<ul class="ul-block">
<li class="list_item">menu1</li>
<li class="list_item">menu2</li>
<li class="list_item">menu3</li>
</ul>
<script src="./main.js"></script>
</body>
</html>
console.dir(document.childNodes)
console.dir(document.childNodes[1].childNodes)
console.dir(document.childNodes[1].childNodes[0].childNodes)
console.dir(document.childNodes[1].childNodes[2].childNodes)
const nodes = document.querySelectorAll('body')
const nodesArray = Array.from( nodes )
// スプレッド構文(展開)
console.log(...nodesArray)
NodeList(2)
0: <!DOCTYPE html>
1: html
length: 2
[[Prototype]]: NodeList
NodeList(3)
0: head
1: text
2: body
length: 3
[[Prototype]]: NodeList
NodeList(9)
0: text
1: meta
2: text
3: meta
4: text
5: meta
6: text
7: title
8: text
length: 9
[[Prototype]]: NodeList
NodeList(8)
0: text
1: comment
2: text
3: h1
4: text
5: ul.ul-block
6: text
7: script
8: text
9: ul.ul-block
length: 10
[[Prototype]]: NodeList
<body>
<!– コメント123 –>
<h1>確認用</h1>
<ul class=”ul-block”>
<li class=”list_item”>menu1</li>
<li class=”list_item”>menu2</li>
<li class=”list_item”>menu3</li>
</ul>
<script src=”./main.js”></script>
</body>
Setから配列生成
Setは、値に順番はなく重複時は値を保持せずに無視されるコレクションになります。
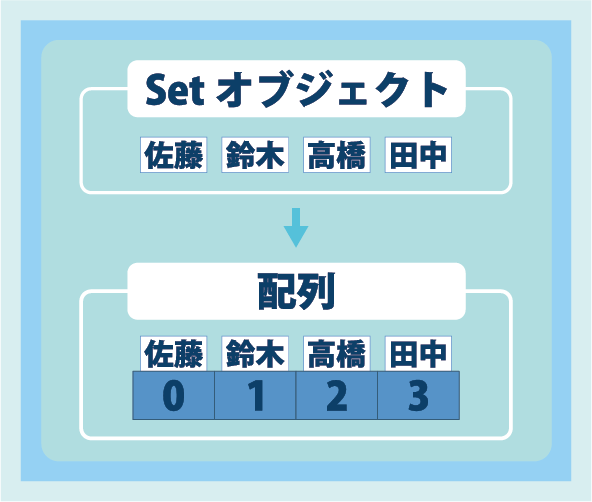
// 重複あり
let names = [ "佐藤", "鈴木", "鈴木", "高橋", "田中"]
// setオブジェクト 重複時に無視される
const namesSet = new Set(names)
// 再度 配列に変換
names = Array.from(namesSet)
console.log("setAry : " + names)
setAry : 佐藤,鈴木,高橋,田中
Mapから配列生成
mapは、キーと値のセットで保存するコレクションです。
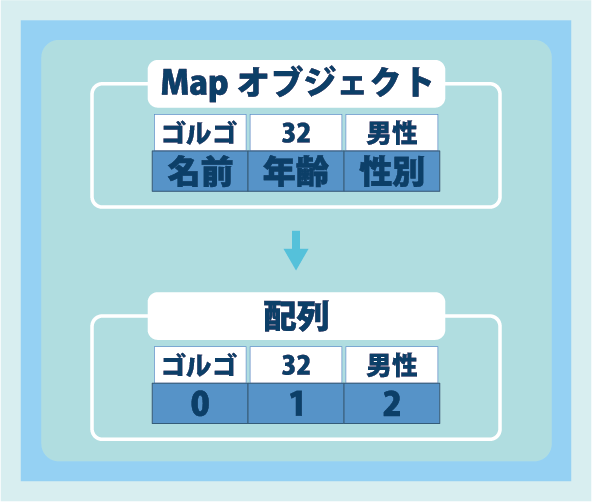
// Mapオブジェクトの生成
const furankenMap = new Map([
["name", "フランケンシュタイン"],
["birth", "1818年3月11日"],
["mail", "franken311@sf.com"],
])
// 配列に変換
furankenAry = Array.from(furankenMap)
valuesAry = Array.from(furankenMap.values())
console.log("furankenAry : " + furankenAry)
console.log("valuesAry : " + valuesAry)
furankenAry : name,フランケンシュタイン,birth,1818年3月11日,mail,franken311@st.com
valuesAry : フランケンシュタイン,1818年3月11日,franken311@st.com
文字列から配列生成
string型の文字列を配列に変換します。
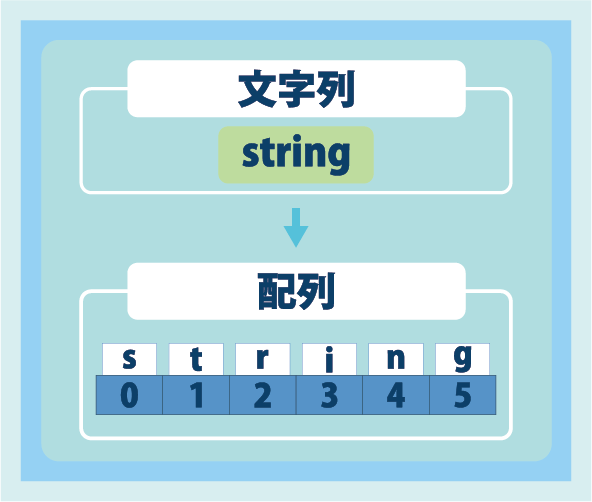
let string = "文字列"
// 配列に変換
stringAry = Array.from(string)
console.log("stringAry : " + stringAry)
stringAry : 文,字,列
of
ofは、引数の数や型にかかわらず新しいArrayインスタンスを生成します。
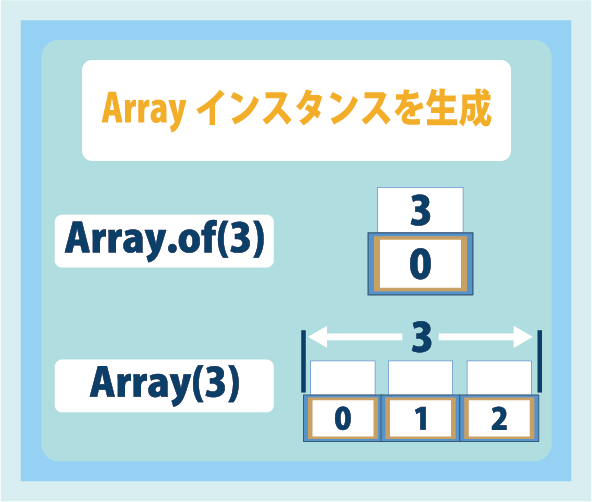
var arrayOf = Array.of(10)
var arrayOfs = Array.of('文字',1,false)
var emptyAry = Array(10)
var ary = Array(1, 2, 3)
console.log("arrayOf : " + arrayOf)
console.log("arrayOfs : " + arrayOfs)
console.log("emptyAry : " + emptyAry)
console.log("ary : " + ary)
arrayOf : 10
arrayOfs : 文字,1,false
emptyAry : ,,,,,,,,,
ary : 1,2,3