記事の内容
v-for
v-forは、配列やオブジェクトをリスト(繰り返し)表示させるディレクティブになります。
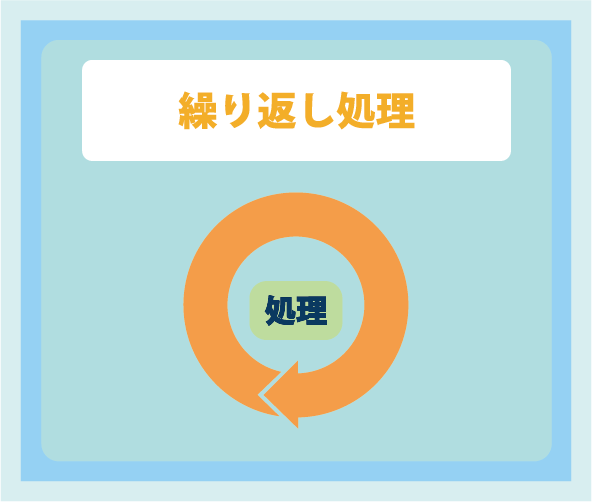
記法
[ HTML ] =============================
<要素名 v-for=”アイテム in リスト”>
<要素名 v-for=”アイテム of リスト”>
※inかofどちらでも良い
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-forについて</title>
</head>
<body>
<div id="app">
<table>
<thead>
<tr>
<th>タイトル</th>
<th>価格</th>
</tr>
</thead>
<tbody>
<tr v-for="book in books">
<td>{{ book.title }}</td>
<td>{{ book.price }}円</td>
</tr>
</tbody>
</table>
</div>
<script src="./js/for.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
books: [
{
title: "HTML & CSS & JavaScript",
price: 2000,
},
{
title: "CSS設計完全ガイド",
price: 3280,
},
{
title: "リーダブルコード",
price: 2400,
},
],
};
},
}).mount("#app");
table {
border-collapse: collapse; /* セルの線を重ねる */
text-align: left;
background-color: white;
}
th,
td {
border: solid 1px; /* 枠線指定 */
}
th {
background-color: #05384b;
color: white;
}
出力結果
タイトル | 価格 |
---|---|
{{ book.title }} | {{ book.price }}円 |
インデックス番号を取得する
v-forでは、アイテム以外にもインデックス情報も取得できます。
記法
[ HTML ] =============================
<要素名 v-for=”( アイテム , インデックス ) in リスト”>
<要素名 v-for=”( アイテム , インデックス ) of リスト”>
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-for indexについて</title>
</head>
<body>
<div id="app">
<table>
<thead>
<tr>
<th>No</th>
<th>タイトル</th>
<th>価格</th>
</tr>
</thead>
<tbody>
<tr v-for="(book,index) in books">
<td>{{ index + 1 }}</td>
<td>{{ book.title }}</td>
<td>{{ book.price }}円</td>
</tr>
</tbody>
</table>
</div>
<script src="./js/index.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
books: [
{
title: "HTML & CSS & JavaScript",
price: 2000,
},
{
title: "CSS設計完全ガイド",
price: 3280,
},
{
title: "リーダブルコード",
price: 2400,
},
],
};
},
}).mount("#app");
table {
border-collapse: collapse; /* セルの線を重ねる */
text-align: left;
background-color: white;
}
th,
td {
border: solid 1px; /* 枠線指定 */
}
出力結果
No | タイトル | 価格 |
---|---|---|
{{ index + 1 }} | {{ book.title }} | {{ book.price }}円 |
オブジェクト取得に関して
オブジェクト取得では、第3引数まで指定できます。
記法
[ HTML ] =============================
<要素名 v-for=”( インデックス , バリュー , キー ) in オブジェクト”>
<要素名 v-for=”( インデックス , バリュー , キー ) of オブジェクト”>
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/main.css" />
<title>v-for indexについて</title>
</head>
<body>
<div id="app">
<ul>
<li v-for="(value, key, index) in TomojiObj">
インデックス:{{ index }}<br />
キー:{{ key }}<br />
値:{{ value }}
</li>
</ul>
</div>
<script src="./js/obj.js"></script>
</body>
</html>
Vue.createApp({
data() {
return {
TomojiObj: {
name: "Tomoji",
height: 170,
weight: 60,
},
};
},
}).mount("#app");
出力結果
-
インデックス:{{ index }}
キー:{{ key }}
値:{{ value }}